Data Size: The sizeof() Function
C++ is a powerful language, and this is no way of speaking.Proof of this is the responsibility that the programmer has with each byte of computer memory through the C++ language programming.
Whenever we declare a variable we are directly reserving bytes from the memory of your machine, and giving access to the address of that placeholder through the variable name.
There is a function (block of code, ready to use) that gives us the size of each type of data we study, is the function: sizeof()
To know the size of a data, just put it in parentheses in the command: sizeof(variable)
And it 'returns' as much bytes occupies.
The program below shows the reserved values for each variable type on my computer:
#include <iostream> using namespace std; int main() { cout << "Variable 'int' occupies : " << sizeof(int) << " byte(s)" << endl; cout << "Variable 'char' occupies : " << sizeof(char) << " byte(s)" << endl; cout << "Variable 'float' occupies : " << sizeof(float) << " byte(s)" << endl; cout << "Variable 'double' occupies: " << sizeof(double) << " byte(s)" << endl; cout << "Variable 'bool' occupies : " << sizeof(bool) << " byte(s)" << endl; return 0; }
Make yours and post in the comments, this can change from one operating system to another.
In my notebook the result was:
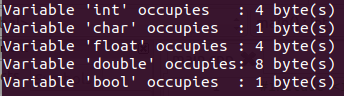
Integer data type: short, long and unsigned
See that an integer occupies 4 bytes.Whether to store the number 0, 1, 2112 or 2147483647, it stores 4 bytes of your computer's memory. But sometimes this can be wasteful.
Let's assume you are working with a microcontroller or want to make the system a digital clock or microwave timer. In these cases, memory is a RARE and LIMITED resource, the less wasted the better.
The solution is instead of declaring 'int variable', do:
short var;
Because it will use less memory space.
What if you're making software for NASA to study planets that are billions of trillion kilometers away and need to use gigantic numbers? Then you use the long:
long var;
Remembering that integers are of two types: negative and positive.
If you are going to work with age, for example, it makes no sense to work with negative numbers, do you agree?
In this case, use unsigned variables:
unsigned var;
The numeric extension of each type is:
short: from -32,768 to +32,767
unsigned short: from 0 to +65,535
int: from -2,147,483,648 to + 2,147,483,647
unsigned int: from 0 to 4,294,967,295
long: from -2.147.483.648 to +2.147.483.647
unsigned long: from 0 to 4,294,967,295
Let's see how much space each of these types occupies?
The code is:
#include <iostream> using namespace std; int main() { cout << "Variable 'short' occupies : " << sizeof(short) << " bytes" << endl; cout << "Variable 'unsigned short' occupies: " << sizeof(unsigned short) << " bytes" << endl; cout << "Variable 'unsigned int' occupies : " << sizeof(unsigned int) << " bytes" << endl; cout << "Variable 'long' occupies : " << sizeof(long) << " bytes" << endl; cout << "Variable 'unsigned long' occupies : " << sizeof(unsigned long) << " bytes" << endl; return 0; }
float and double precision
Since we're talking about integer variables, their range, size, and precision, let's revisit the float and double variables used to represent decimal numbers:- float: single precision, occupies 4 bytes and goes from 3.4 x 10 ^ (- 38) to 3.4x10 ^ 38
- double and long double: double precision, occupying 8 bytes and ranging from 1.7 x 10 ^ (- 308) to 1.7x10 ^ 308
No comments:
Post a Comment