Storing Information
One of the pillars of computing is the storage of information, data.The computer basically does two things: calculations and data storage.
Almost always, it does both: data processing, like store, change, delete, do math operations, and so on.
In this tutorial, although long is quite simple, we will introduce the concepts of data types and variables in C ++, highly important knowledge for working with computer programming.
Let us know the most varied types of data, their characteristics and functions.
Beforehand, know that every variable you will use to store data throughout your programs must be declared in advance.
Using a variable that has not been declared will result in errors at compile time.
The integer data type: int
This datatype is specifically for storing numeric data of the integer type.The integers are:
- ..., -3, -2, -1, 0, 1, 2, 3, ...
Let's now create and declare a variable called age:
- int age;
Ready. At this point, the computer will take a place from your computer's memory and set it aside for the age variable. And what is stored? Initially, 'junk'.
Let's now assign the value 18 to this variable:
- age = 18;
Now, whenever we use the number variable, it will be replaced by the integer 18.
Look:
#include <iostream> using namespace std; int main() { int age; age = 18; cout << "My age is: "<< age <<endl; return 0; }
Note that cout does not print the word 'age', but the value contained within this variable.
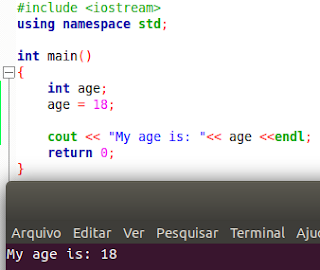
Character data type: char
In addition to integers, we can also store characters, ie letters.For this, we use the keyword char (from character).
Let's declare a char variable, named letter.
- char letter;
Now let's assign a value to it. In this case, as it is a char, we must store some letters of the alphabet, such as C:
- letter = 'C';
Note that characters must be enclosed in single quotes.
Let's print this letter on the screen:
#include <iostream> using namespace std; int main() { char letter; letter = 'C'; cout << "The third letter of the alphabet is: "<< letra << endl; return 0; }
Remembering that 'a' is different from 'A', are two distinct characters.
Characters are most commonly used together, forming text. We will study this in a later section, about Strings, in our course.
Understand:
- 5 - is a number, a data of type int
- '5' - is a character, a letter, cannot be used for example in mathematical operations
Floating Data Type: float e double
We have already learned to deal with integers.However, not everything in life is an integer, like your age.
Often we need to work with fractional values, decimal values.
For this we use the float and double data types:
- float price;
- double value;
The difference is that float has single precision, and double has double precision (that is, it fits a larger fractional part, and a larger number of bytes of memory has been reserved for this type of variable).
Let's look at a use:
#include <iostream> using namespace std; int main() { float price; price = 14.99; cout << "Progressive C++ e-book costs: $ "<< price << endl; return 0; }
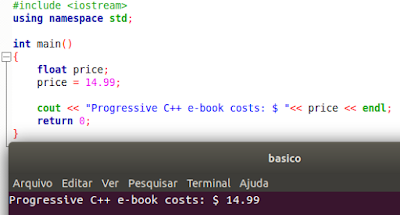
The Boolean data type: bool
You may have heard that in computing (or technology in general) it's all 1 or 0, isn't it?In fact, the values 1 and 0 are very important because they represent true and false, consecutively.
There is a data type for storing only true / false information (called booleans), bool.
Declaring:
- bool true;
Assigning a boolean value:
- truth = true;
Displaying the value of true on screen:
#include <iostream> using namespace std; int main() { bool truth; truth = true; cout << "Truth in C++ is: "<< truth << endl; return 0; }
Results:
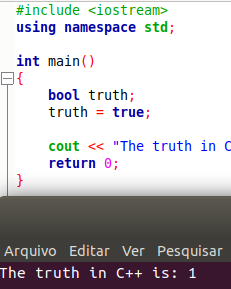
Exercises:
1. Make a C++ program that displays the value of two Boolean variables, showing each possible assigned value. Use two variables.2. Redo the previous exercise, now declaring only one variable.
Variables names
Since we have full power to choose the name we want for our variables, we also have some responsibilities.The first is not to choose keywords, which will be lists in the following topic.
Another responsibility is that of the organization.
You will be tempted to use:
int a, float b, char c
Avoid these names. Use:
int age;
float diameter;
char letter;
That is, variable names that mean something related to the value you will store there. This helps a lot when your programs get bigger and more complex.
Also avoid:
double salaryprogrammer;
Use:
double salary_programmer;
Or yet:
double salaryProgrammer;
Notice how this makes it easier to read and from the start we can already predict what kind of information you have in these variables, do you agree?
Reserved Keywords
There are some words you should not use as names for your variables, as they are reserved for the inner workings of C ++.Are they:
and, and_eq, asm, auto, bitand, bitor, bool, break, case, catch, char, class, compl, const, canst cast, continue, default, delete, do, double, dynamic_ cast, else, enum, explicit, export, extern, false, float, for, friend, goto, if, inline, int, long, mutable, namespace, new, not, not_ eq, operator, or, or_eq, private, protected, public, reg i ster, reinterpret_cast, return, short, signed, sizeof, static, static_cast, struct, switch, template, this, throw, true, try, typedef, typeid, typename, union, unsigned, us ing, virtual, void, volatile, wchart, while, xorxor_eq
Exercise Answer
#include <iostream> using namespace std; int main() { bool booleanValue; booleanValue = true; cout << "Truth in C++: "<< booleanValue << endl; vabooleanValuelorBooleano = false;
cout << "False in C++: " << booleanValue << endl; return 0; }
No comments:
Post a Comment