Doing calculations, counting ... is the basis of computing, and hence of programming.
In this tutorial, we will learn how to do C ++ accounts.
C++ sum: + operator
To perform the addition operation, we use the + operator between two values.It can be in literal form: 1 + 1
Or values stored in variables: value1 + value2
A program that shows the sum of the numbers 21 and 12:
#include <iostream> using namespace std; int main() { cout << 21 + 12; return 0; }

These values could be previously stored in variables, var1 and var2, for example and summed later, see:
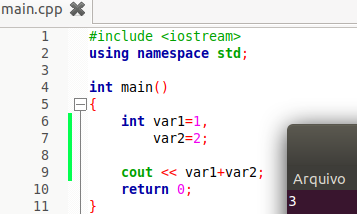
Notice how we declare the variables var1 and var2.
Since both are integers, we do not need to do:
int var1=1;
int var2=2;
We can 'summarize', write less and do:
int var1=1,
var2=2;
Much more fancy, isn't it?
C++ Subtraction: - operator
As we add, we can subtract, and for that, just use the - (minus) operator.Let's subtract 21 from 12, and then do 1 minus 2:
#include <iostream> using namespace std; int main() { int var1=1, var2=2; cout << "21 - 12 = " << 21-12 << endl; cout << "1 - 2 = " << var1-var2; return 0; }
See the resulto:
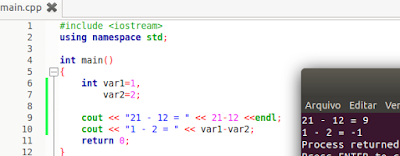
Here we use strings:
"21 - 12 = "
"1 - 2 = "
Here, numbers, math values:
21 - 12
var1 - var2
It's two different things, it's two different data types.
We did this and put everything together in the cout command (simple C++ output), to make the output very cute and neat.
C++ multiplication: * operator
In our day to day, to describe the multiplication operation, we use the x symbol, sometimes, true?However, in C++ programming, 'x' is a letter.
To identify the product operator, we use the asterisk: *
So to multiply 2 by 2, or 21 by 12, we do:
#include <iostream> using namespace std; int main() { int var1=2, var2=2; cout << "2*2 = " << var1 * var2 <<endl; cout << "21 * 12 = " << 21*12; return 0; }
Compilation result:

Note that we declare the variables all on the same line. We did this because they are the same type, the integer type, and separated by comma.
We could also have done:
int var1 = 2;
And then: var1 * var1
After all, are of equal values, agree?
C++ division: / operator
In daily life, the division operator is also different, it is the “÷”.In programming, the symbol is: /
Let's divide 4 by 2, and then 1 by 3.
The code is:
#include <iostream> using namespace std; int main() { float var1, var2; var1=1; var2=3; cout << "4 / 2 = " << 4/2 <<endl; cout << "1 / 3 = " << var1/var2; return 0; }
The compilation results in:
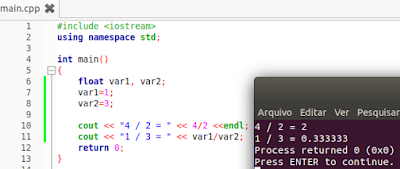
Note that we first declare the variables:
float var1, var2;
And only then we initialize with the values 1 and 3:
var1 = 1;
var2 = 3;
It is another way of declaring and initializing variables.
In the first cout, we divided 4 by 2. Two integers, with results of integer division, and C++ showed the resulting integer: 2.
But by dividing 1 by 3, the result is decimal. So we declared the variables var1 and var2 as float, because we knew we would need the decimal places to represent the division operation.
Change the float declaration to double, what happened? Say it in the comments.
Change the float declaration to int, what happened? Say it in the comments.
Remainder: % operator
Finally, let's use the% arithmetic operator, called the modulus or remainder of the division.
Let's go back to school and remember how we used to contain:
See that 'remainder'? It is the rest of the division of 17 by 2.
To get this result, do:
- 17 % 2 = 1
This operator is special because it's only used with integers, ok?
In the future, in our C++ course, we will use the module operator (or remainder of the division) for some specific algorithms, such as finding prime numbers and working with multiples.
Math exercises in C++
01. Write a program that adds the numbers 10, 20 and 30
02. Write a program that divides 21 by 12
03. What is the module, or remainder of the division, of number 21 by 12?
04. What is the error of the code below?
#include <iostream> using namespace std; int main() { number = 2112; int number; cout << "Number: "<< number <<endl; return 0; }
05. Store in the 'sum' variable the result exercise 1. Then divide by 3 to find the average, what value did you find?
06. Redo the previous exercise, but without using any variables, that is, print the value of the direct result in cout.
07. If you do: 10 + 20 + 30/3, what is the result? Is it the average? If not, why did it go wrong?
Soon, still in our Introduction to C++ section, let's return to the operators in relation to their precedence.
No comments:
Post a Comment