Pass by Value
We have already learned how to send information to functions through arguments and parameters.And we saw that this pass is called pass by value, because we only pass the value to the function.
If we send a variable to a function, and within that function that variable is changed, it is not changed outside the function. Test the following code, which squares a number:
#include <iostream> using namespace std; void square(int num); int main() { int number = 6; cout<<"Before : num = "<<number<<endl; square(number); cout<<"After : num = "<<number<<endl; return 0; } void square(int num) { num = num*num; cout<<"During : num = "<<num<<endl; }When we invoke the function: square (number), we are actually passing a copy of the value of the variable number.
Within the function, the num variable will receive a copy of the value of number. That is, it will not have access to the original variable number, only its value! Therefore, passing by value.
Reference Parameter: &
Be the variable declaration:- int num = 6;
What we are doing is allocating, reserving, a space in the memory of your computer.
So when we use num, C++ understands that we must go to the address this variable points to and get the number inside that memory location.
As we have seen, when we pass this normal variable to a function that expects a normal variable, it takes only a copy of its value, and does not mess with the original content.
However, there is another special type of variable, the reference variable.
It is special because if we pass a variable to a function and the function wants to get the reference variable through a reference parameter, it will have access to the memory address (the reference) where this variable is originally stored.
That is: we will actually have access to the variable, not just its copy.
To get the reference of a variable, just use the & operator before the variable name, in the function parameter! It is the reference parameter. Both in the prototype and in the function declaration.
So the previous code example looks like this:
#include <iostream> using namespace std; void square(int &); int main() { int number = 6; cout<<"Before : num = "<<number<<endl; square(number); cout<<"After : num = "<<number<<endl; return 0; } void square(int &num) { num = num*num; cout<<"During : num = "<<num<<endl; }See now the result:
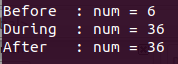
In fact, the function was able to change the value of the variable because this argument pass to the parameter was by reference parameter.
What happens here is that the parameter will not capture the value of the argument but its reference to where it is pointing in memory. Speaking of pointing to an address, we will study more about it in the pointers and arrays (vectors) sections in C++, where we will study about passing by reference using pointers.
More about C++ Reference Parameters
Some programmers also prefer to declare the prototype like this:- square (int&);
You can also use it like this in the prototype:
- square(int &num);
- square(int& num);
Also, the important thing is that & is in both the prototype and function declaration, ok?
Remember that if your parameter is reference, it can only work with reference variable.
It would be a mistake if you use an argument that is not a variable, such as a literal, an expression, or a constant, for example:
- square(10); // must use square (number);
- square(number+1); // it's an expression, avoid
When we do: square (int &num)
Read: "num is a reference to an integer", that is, it is referencing, pointing, indicating a memory location where an integer is stored. Since you have the location of the original variable, you can change this value.
Read: "num is a reference to an integer", that is, it is referencing, pointing, indicating a memory location where an integer is stored. Since you have the location of the original variable, you can change this value.
Value passing and reference exercise
Create a program that prompts the user for an integer. Then it must turn this value into its cube. Do this by using a function that receives pass by value and another that uses parameter and reference variable. Make it clear that one changes the value only within the function (value) and the other changes the original value of the variable (reference).
Paste in the comments, your solution.
Paste in the comments, your solution.
No comments:
Post a Comment