Local variable
When we declare a variable within a function, we say that it is local.This is due to the fact that it 'exists' only for those who are within the function, that is, only inside can see it and its value.
Other commands in other functions cannot access it normally.
To illustrate this, let's declare the variable myVar with value 1, inside main().
Next, let's call the print () function, which will print the value of myVar:
#include <iostream> using namespace std; void print() { cout<<myVar; } int main() { int myVar=1; print(); return 0; }This code is neither compiled, the error appears:
"'myVar’ was not declared in this scope | "
That is, the myVar variable is not within the scope of the print() function, it is as if it did not exist.
The opposite also applies:
#include <iostream> using namespace std; void print() { int myVar=1; } int main() { cout<<myVar; return 0; }That is, not even the main() function can see the internal content of the print() function.
Now let's declare myVar both inside print() and inside main(), and let's print both values:
#include <iostream> using namespace std; void print() { int myVar=1; cout << "In print() = "<<myVar<<endl; } int main() { int myVar=2; cout << "In main() = "<<myVar<<endl; imprime(); return 0; }Results
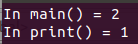
That is, we can declare variables of the same name, but in different functions.
And the functions only 'see' what's inside that function, ok?
Global variable
There is a way to make the same variable seen and accessed by several functions, just make it a global variable.To do so, simply declare it outside the scope of functions.
For example, let's declare the variable pi, and store the value of pi.
Next, we will ask the radius value from the user, and using two other functions, we calculate the perimeter and the area of the circle:
#include <iostream> using namespace std; float pi = 3.14; float perimeter(float r) { return 2*pi*r; } float area(float r) { return pi*r*r; } int main() { float rad; cout<<"Radius: "; cin>>rad; cout<<"Perimeter: " << perimeter(rad)<<endl; cout<<"Area : " << area(rad)<<endl; return 0; }Note that we use the pi variable in functions, without declaring them within their scope.
If we were to use local variables, we would have to declare pi more than once, which would not be very efficient.
Use global variables when they are needed in many different parts of your code.
And you can use the same name for a local and global variable. In this case, the local variable will have priority.
Global constants
Very careful when using global variables. In complex programs, it is easy to 'lose control' of global variables, as they can be changed anywhere in code.In most cases, give priority to using same arguments.
However, if you want to use global variables that should not be changed, use the const keyword before declaring the variable:
- const float pi = 3.14;
Let's suppose a hacker broke into your system, and entered a function called change(), which will change the value of pi to 4, sabotaging your project:
#include <iostream> using namespace std; const float pi = 3.14; void change() { pi = 4; } int main() { change(); return 0; }As the variable was declared with the const keyword, it will give the error:
"| 7 | error: assignment of read-only variable pi ’|"
That is, the variable is read only, cannot change the 'pi'. And in fact, no program should change pi, so it makes sense that it is global and constant, do you agree?
Let's assume that you will create a system for a large supermarket chain, and you will set the discount price at 10%, do:
- const float off = 0.1;
Ready. Now thousands of functions can access the discount amount.
What if you want to increase the discount to 20%?
Easy, just do:
- const float off = 0.2;
See that you changed just one thing, just one variable. But it automatically modified the calculations of the thousands of functions that use this variable directly.
It changes only once, and the change occurs in multiple corners.
If you had done it locally, you would have to go to each function and change variable by variable ... it would take hours or days.
But with constant global variable, no. Changes only once. And everything changes.
Did you get the utility of global and constant variables?
Static local variable
When we declare a variable within a function, it is created and reserved in computer memory at the beginning of the function and is destroyed at the end of the function's execution.In the code example below, we initialize the myVar variable to 1, print, increment by one, and terminate the function.
#include <iostream> using namespace std; void test() { int myVar=1; cout<<myVar<<endl; myVar++; } int main() { test(); test(); return 0; }We call the test() function twice, and what appears on the screen is always the same: the value 1.
Each time the function runs, the variable is created, initialized and the value 1 is displayed. It is incremented, but then the function ends and the variable simply dies.
We then say that local variables are nonpersistent. That is, they do not 'persist', they are created and destroyed along with the function.
There is a way to make the variable persistent, that is, it is created initially and not destroyed, its address and value in memory still remains. These are the static variables.
To declare a variable to be static, just use the static keyword before the declaration:
- static int myVar;
Veja:
#include <iostream> using namespace std; void test() { static int myVar=1; cout<<myVar<<endl; myVar++; } int main() { test(); test(); test(); test(); return 0; }Ready. No matter how many times you call the test() function, the myVar variable you will use is declared and initialized only once. When the function ends, it will still persist and when calling the function again, it will already take the previous value of the variable, to print and increment.
Like global variables, local static are always initialized to 0 if you do not explicitly initialize. And if you boot, this boot will only occur once, as you saw in the previous code example, ok?
No comments:
Post a Comment