Simple Arithmetic Average in C++
The most basic average of all is the so-called simple arithmetic, where you basically add up all the terms and divide by the total of terms.To average two numbers:
(a + b) / 2
From three numbers:
(a + b + c) / 3
From four numbers:
(a + b + c + d) / 4
From n numbers:
(a + b + c ...) / n
Simple Arithmetic Average Exercises
- "Create a program that asks the user for two grades, and returns their average."
Our code is:
#include <iostream> using namespace std; int main() { float grade1, grade2, average; cout << "Grade 1: "; cin >> grade1; cout << "Grade 2: "; cin >> grade2; average = (grade1+grade2)/2; cout << "Average: " << average; return 0; }
Note that while it is a very simple formula, it is good practice to put the sum within parentheses to avoid making mistakes like:
a + b/2
(In this case, we would be adding a with b/2)
- "Do the same as the previous exercise, but for 3 grades."
Our code:
#include <iostream> using namespace std; int main() { float grade1, grade2, grade3, average; cout << "Grade 1: "; cin >> grade1; cout << "Grade 2: "; cin >> grade2; cout << "Grade 3: "; cin >> grade3; average = (grade1+grade2+grade3)/3; cout << "Average: " << average; return 0; }
Weighted Average with C++
In the arithmetic average, all terms have the same 'weight', that is, they contribute equally to the final value of the average.In the weighted, each term has a weight, see the formula:
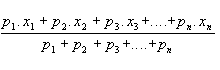
The terms are x1, x2, x3, ...
The respective weights are p1, p2, p3, ...
In this case, summing all terms multiplied each by their weight, and we divide by the sum of the weights.
- "The MIT entrance exam has a weight of 3 for Mathematics, 2.5 for Physics, 2.5 for Chemistry, 1.0 for Portuguese and also 1.0 for English. Create a system that asks for user grades and returns their average."
Look our code:
#include <iostream> using namespace std; int main() { float math, phy, chem, port, eng, average; cout << "Math grade "; cin >> math; cout << "Physics grade: "; cin >> phy; cout << "Chemistry grade: "; cin >> chem; cout << "Portuguese grade: "; cin >> port; cout << "English grade: "; cin >> eng; average = (3*math + 2.5*phy + 2.5*chem+port+eng) / 10; cout << "Average: " << average; return 0; }
Note that the sum of the weights is 10.
Average Exercises in C++
Solve the exercises below and post your solutions in the comments.01. Solve the previous exercises, now without using the average variable.
02. Make a program that receives the amount of gallons a person has fueled in the car and the amount of miles they have traveled with that fuel, then calculate the average (ie how many miles per kilometer) they make.
03. Make a program that asks for the size of a downloadable file (in MB) and the speed of an Internet link (in Mbps), calculate and enter the approximate file download time using this link (in minutes).
04. A new super-economical car model has been launched.
He makes 20 km with 1 liter of fuel.
Each liter of fuel costs $ 5.00.
Make a program that asks the user how much money they have, and then tells how many gallons of fuel they can buy and how many miles the car can handle on that much fuel.
Your script will be used on the car's onboard computer.
No comments:
Post a Comment