Before you see the code, try to solve it.
It must receive two user numbers, and display the operations of:
- Sum
- Subtraction
- Multiplication
- Division of the first by the second
- Rest of the division of the first by the second
- Percentage of the first relative to the second
- Arithmetic average
Your program should look like:
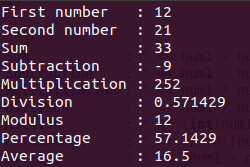
Try it!
How to make a calculator in C++
See how our code looked:Yours, how was it? Different?#include <iostream> using namespace std; int main() { float num1, num2; //Receiving data cout << "First number : "; cin >> num1; cout << "Second number : "; cin >> num2; //Displaying operations cout << "Sum : " << num1 + num2 << endl; cout << "Subtraction : " << num1 - num2 << endl; cout << "Multiplication : " << num1 * num2 << endl; cout << "Division : " << num1 / num2 << endl; cout << "Modulus : " << (int)num1 % (int)num2 << endl; cout << "Percentage : " << 100.0*(num1/num2) << endl; cout << "Average : " << (num1 + num2)/2 << endl; return 0; }
Put it in the comments!
See that the only thing different was the excerpt:
(int) num1% (int) num2
This is called casting, that is, since the module operator (rest of division) only makes sense with integer values and our variables are float, we put (int) before the variables to tell C ++ that we want to handle those variables, at that time, as integers.
No comments:
Post a Comment