In this tutorial from our C++ e-book, we will learn how to create an array of objects, as well as how to manipulate them.
Creating an array with Objects in C++
In the past tutorial, we created a class that averaged two or three grades for students. We will do something similar in this tutorial.
Let's create the Student class, and initially it gets two grades. The constructor function correctly sets variables, and has a function called getAverage() that returns the average of the two grades:
#include <iostream> using namespace std;class Student { private: double grade1, grade2; public: Student(); Student(double, double); double getAverage(); }; Student::Student() { grade1 = 0; grade2 = 0; }double Student::getAverage() { return (grade1+grade2)/2; } int main() { Student NeilPeart(10, 8); cout<<NeilPeart.getAverage()<<endl; return 0; }
Note that we created only one object, the student Neil Peart. But which school has only one student? There are thousands, but let's take it easy. We will now work with 5 students. We could do:
- Student alu1, alu2, alu3, alu4, alu5;
But, what if it was a real project, with a thousand students? Would you do it up to the alu1000?
Of course not, right, buddy!
You studied by Progressive C++, you are a smart person and you will soon remember our beloved and beautiful arrays, to work with many variables at the same time.
To declare 5 integers, we do:
- int num[5];
To declare 5 chars, we do:
- char letter[5];
So guess what we do to create 5 objects of the Student class? That's right:
- Student alu[5];
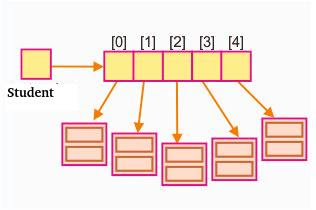
#include <iostream> using namespace std; class Student { private: double grade1, grade2; public: Student(); Student(double, double); double getAverage(); }; Student::Student() { grade1 = 0; grade2 = 0; } Student::Student(double g1, double g2) { grade1 = g1; grade2 = g2; } double Student::getAverage() { return (grade1+grade2)/2; } int main() { Student alu[5]; cout<<alu[0].getAverage()<<endl; return 0; }
How to Initialize Objects from an Array
Student alu[5]; alu[0] = Student(10,9); alu[1] = Student(10,8); alu[2] = Student(10,7); alu[3] = Student(10,6); alu[4] = Student(10,5);
Student alu[5] = { Student(10,9), Student(10,8), Student(10,7), Student(10,6), Student(10,5) };
Student alu[5] = { {10,9}, {10,8}, {10,7}, {10,6}, {10,5} };
Accessing members of the Object Array
- name.var
- name.func()
- arr[0].grade
- arr[1].getAverage();
#include <iostream> using namespace std; class Student { private: double grade1, grade2; public: Student(); Student(double, double); double getAverage(); }; Student::Student() { grade1 = 0; grade2 = 0; } Student::Student(double g1, double g2) { grade1 = g1; grade2 = g2; } double Student::getAverage() { return (grade1+grade2)/2; } int main() { Student alu[5] = { {10,9}, {10,8}, {10,6}, {10,5} }; cout<<alu[0].getAverage()<<endl; cout<<alu[1].getAverage()<<endl; cout<<alu[2].getAverage()<<endl; cout<<alu[3].getAverage()<<endl; cout<<alu[4].getAverage()<<endl; return 0; }
No comments:
Post a Comment