First, let's review a little of what we learned in the Functions section.
Passage by Value and Reference Variable
Run the following program, which squares a number entered by the user.It shows the number entered, it squared and then the original value, again:
#include <iostream> using namespace std; int square(int num) { num = num*num; cout << "Squared: " << num <<endl; } int main() { int num; cout <<"Type a number: "; cin >> num; cout <<"Number typed: " << num << endl; square(num); cout <<"num value: " << num << endl; return 0; }
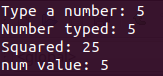
See that we pass the variable 'num' to the function, and inside it is squared. We show this value within the function, and then show it again when the function call is finished.It did not change the value stored in 'num'.
This is because when we pass a variable to a function, the function makes a copy of its value. It will not use the variable directly, but its value only, so the original variable is not changed.
To give access directly to the variable, we can use the reference variables.
Which is basically passing the name of the variable, with the symbol & in front, see how it looks:
#include <iostream> using namespace std; int square(int &num) { num = num*num; cout << "Squared: " << num <<endl; } int main() { int num; cout <<"Type a number: "; cin >> num; cout <<"Number typed: " << num << endl; square(num); cout <<"num value: " << num << endl; return 0; }
Now notice that the value of 'num' directly has been changed inside the square() function:
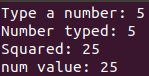
This happened because we used the reference variable &num instead of just num.
And now that you've studied pointers and memory addresses, you know that by using & we are dealing directly with memory addresses. That is, the function will now have access to the memory address of variable num and will change that block of memory directly.
How to Use Pointers in Functions in C++
Another way to change the value of a variable, passing it to a function, is using pointers. In fact, it is important to know how to use this technique, as it is very useful in functions that deal with Strings and in several C++ libraries, for example.Let's create a function, called doub(), that will double the value it receives, using pointers:
Its parameter is: int*#include <iostream> using namespace std; int doub(int*); int main() { int num; cout <<"Type a number: "; cin >> num; cout <<"Number typed: " << num << endl; doub(&num); cout <<"num value: " << num << endl; return 0; } int doub(int *ptr_num) { (*ptr_num) = (*ptr_num) * 2; cout << "Double: " << *ptr_num <<endl; }
That is, it expects an int pointer. If it expects a pointer, we have to pass as a ... memory address! So we did: doub(&num);
Remember: a pointer is a variable that stores a memory address!
Within the function, 'ptr_num' is a pointer. Pointer to the memory address of the 'num' variable in the main() function.
We want to double the amount it points to. Now, how do you access the value stored in the location to which the pointer points? Using asterisk: *ptr_num
So, to double the value of the variable 'num', using the pointer 'ptr_num' that points to it, just do:
*ptr_num = *ptr_num * 2;
In order not to get confused with the operators, you can do it as soon as it gets more organized:
(*ptr_num) = (*ptr_num) * 2;
The power of pointers
A great advantage of working with pointers is that they can deal in a more 'global' way, so to speak, with the variables they point to.For example, note that we always ask the user for a number within the main() function, and from there we send that value or its address to the most diverse functions.
Using pointers, we can get user values inside a function, like getNum(), see:
We declare the variable 'num', and pass its address to the doub() variable, this address will be stored inside the ptr_num pointer.#include <iostream> using namespace std; void getNum(int *); int doub(int*); int main() { int num; getNum(&num); cout <<"Number typed: " << num << endl; doub(&num); cout <<"num value: " << num << endl; return 0; } void getNum(int *ptr_num) { cout <<"Type a number: "; cin >> *ptr_num; } int doub(int *ptr_num) { (*ptr_num) = (*ptr_num) * 2; cout << "Double: " << *ptr_num <<endl; }
Now, we want to change the value of ptr_num, so just use: *ptr_num
And ready, magically, the variable that was declared there in the main() function was changed inside the getNum() function.
Powerful, these pointers, right?
No comments:
Post a Comment