Arrays and Pointers, Pointers and Arrays
Just for curiosity, let's declare an array of integers, named 'numbers', initialize and then simply print the value '*numbers':#include <iostream> using namespace std; int main() { int numbers[]={1, 2, 3, 2112}; cout << *numbers << endl; return 0; }Look how interesting the result:
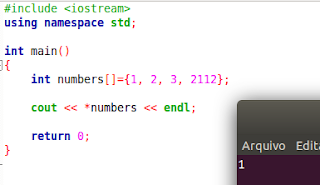
That is: the name of the array works like a pointer.
And where does it point? For the first element of the array.
So, whenever we have an array named: arr
If we use the name of the array variable, it will behave like an array that points to: arr[0]
And to point to the other members of the array?
Remember the following rule:
- arr[index] = *(arr + index)
arr[0] can be referenced by *(arr + 0)
arr[1] can be referenced by *(arr + 1)
arr[2] can be referenced by *(arr + 2)
...
arr[n] can be referenced by *(arr + n)
We can make a pointer named 'ptr' point to the first element of an array in the following ways:
- int *ptr = numbers;
- int *ptr = &numbers[0];
Let's print an entire array, using just one pointer:
#include <iostream> using namespace std; int main() { int numbers[6]={1, 2, 3, 4, 5, 2112}, *ptr = numbers; for(int aux=0 ; aux<6 ; aux++) cout << *(ptr+aux) << endl; return 0; }
Pointer Arithmetic
In the previous C++ code example, you saw that we did an add operation with pointers several times: ptr + aux, where aux is an integer variable from 0 to 5, to go through the array.We could have done the same program with the ++ operator, see:
#include <iostream> using namespace std; int main() { int numbers[6]={1, 2, 3, 4, 5, 2112}, *ptr = numbers; for(int aux=0 ; aux<6 ; aux++){ cout << *ptr << endl; ptr++; } return 0; }That is, each time we add a unit to the pointer (++), it points to the next element in the array. We can do the reverse, point to the last element of the array and go on decrementing:
#include <iostream> using namespace std; int main() { int numbers[6]={1, 2, 3, 4, 5, 2112}, *ptr = &numbers[5]; for(int aux=5 ; aux>=0 ; aux--){ cout << *ptr << endl; ptr--; } return 0; }We could also use the operators -= or +=
The logic is as follows.
When we point the pointer to the array, it will point to the memory address of the first element. Let's assume that it is the 1000 memory block.
When we do: ptr++
It does not increment the memory address by one, it does not go to 1.
It goes to: 1000 + sizeof(int)
As the array is of integers, each block of the array occupies 4 Kbytes, so ptr will point to address 1004. Then to block 1008, then 1012 ...
If the array were double, it would point to the addresses: 1000, 1008, 1016, 1024 ... every time we incremented, since the double variable occupies 8 Kbytes ( sizeof(double) ).
See how is the code of the program that prints only the elements of even index, of the array (0, 2 and 4):
#include <iostream> using namespace std; int main() { int numbers[6]={1, 2, 3, 4, 5, 2112}, *ptr = numbers; for(int aux=0 ; aux<3 ; aux++){ cout << *ptr << endl; ptr += 2; } return 0; }This is the logic and mathematics of the pointers. That is why it is so important to define the type of pointer (int, char, double ...), since the pointers point to entire blocks of memory, the size of these blocks varies according to the type of data.
No comments:
Post a Comment