- What is a function?
- What is a function for?
- How to create one?
- How to use a function?
C++ Function: What It Is and What It Is For
Have you ever seen a board with some hardware, like your computer board, or the inside parts of a television, cell phone ... it's something like this:
See: There is not just one piece responsible for doing everything.
A board is a portion of small pieces doing small, very specific jobs.
Because they do very specific things, such as counting or measuring temperature, for example, we can use these little pieces on other boards, such as a car or a refrigerator.
That is, each little piece has a specific function, does something.
In C++ programming, it's the same thing: Function is a piece of stuff that does a specific thing.
Putting it best, function is a block of code, with some C++ commands inside, that does a certain task. A function can receive and return information for the caller.
It is much better, more efficient, faster, and more feasible to create a board with several small pieces, doing specific things, than a big and only piece doing something alone.
Likewise, it is not feasible to have a single block of giant code, thousands of lines, because it would be extremely difficult to work with, knowing where there are errors, where is something ... the ideal is to go breaking your program in smaller programs that make things more specific and easier to understand.
It is as if each function were a little lego. You put the pieces together and you assemble great and cool things. Your operating system, for example, works like this.
Let's learn how to create a function?
For example:
Within the parentheses, they can receive information to work with these data, are the parameters:
If the function returns any data type, we use return within the code block to return the data type we declared in the function header.
Let's create the hello () function, it returns no data and receives no information, just prints hello, world! on the screen:
To call the function we created earlier, we simply do:
hello();
Ready. C++ goes there to get this function and execute the code that has it, see:
You can invoke (call) function 1, 10 or a million times, just do: hello() ;, without having to repeat the code 1 million times, just reuse using functions.
The menu() displays a menu of options about which time of day you are.
In welcome(), the user will have to type some option, which will be stored in the op variable, declared within the function.
Then, still within this function we make a treatment to display the message correctly:
main() is a special function of C ++, it is automatically executed when we compile and execute our code. Inside it, we first call menu() and only when it finishes executing, it calls welcome().
Note that only after the user enters the value in welcome() and she greets you, will it end and return to main(), which displays a final closing cout.
So far in our course we have done small and simple code examples.
But when you become a professional programmer, it is normal to make systems with hundreds or thousands of lines of code, and that's when you will understand how important the concept of functions is in C++.
You should get into the habit of creating small, simple, easy-to-understand and easy-to-use functions, because whenever you start a new project, you'll use your old functions and existing C++ functions by default.
This technique is called divide and conquer, and throughout our C ++ course you will see that a large and complex program is nothing more than a bunch of small, simple, correctly connected programs that work in harmony.
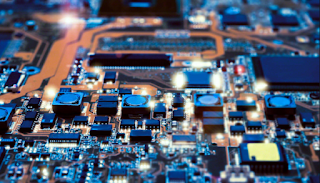
See: There is not just one piece responsible for doing everything.
A board is a portion of small pieces doing small, very specific jobs.
Because they do very specific things, such as counting or measuring temperature, for example, we can use these little pieces on other boards, such as a car or a refrigerator.
That is, each little piece has a specific function, does something.
In C++ programming, it's the same thing: Function is a piece of stuff that does a specific thing.
Putting it best, function is a block of code, with some C++ commands inside, that does a certain task. A function can receive and return information for the caller.
It is much better, more efficient, faster, and more feasible to create a board with several small pieces, doing specific things, than a big and only piece doing something alone.
Likewise, it is not feasible to have a single block of giant code, thousands of lines, because it would be extremely difficult to work with, knowing where there are errors, where is something ... the ideal is to go breaking your program in smaller programs that make things more specific and easier to understand.
It is as if each function were a little lego. You put the pieces together and you assemble great and cool things. Your operating system, for example, works like this.
Let's learn how to create a function?
How to create a function in C++
The code syntax for creating a function is as follows:type name(parameter_list) { // Code of // funtion return something; }Functions may or may not return some information, such as an integer or a float.
For example:
- int function1 () {...} - returns an integer
- float function2 () {...} - returns a float
- void function3 () {...} - returns nothing
Within the parentheses, they can receive information to work with these data, are the parameters:
- void function1 (int num) {...} - Send an integer to the function and return nothing
- int function2 (int num1, float num2) {...} - Send two numbers as a parameter, an integer and a float, and return an integer.
If the function returns any data type, we use return within the code block to return the data type we declared in the function header.
Let's create the hello () function, it returns no data and receives no information, just prints hello, world! on the screen:
void hello() { cout <<"Hello, world!\n"; }
How to call and use a function in C++
To call the function simply write its name, followed by parentheses and the information it should receive.To call the function we created earlier, we simply do:
hello();
Ready. C++ goes there to get this function and execute the code that has it, see:
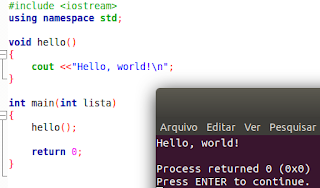
Example of Using C++ Functions
Let's create two functions now.The menu() displays a menu of options about which time of day you are.
In welcome(), the user will have to type some option, which will be stored in the op variable, declared within the function.
Then, still within this function we make a treatment to display the message correctly:
#include <iostream> using namespace std; void menu() { cout<<"What time of day is:\n"; cout<<"1. Morning\n"; cout<<"2. Afternoon\n"; cout<<"3. NIght\n"; } void welcome() { int op; cin>>op; if(op==1) cout<<"Good morning!\n"; else if(op==2) cout<<"Good afternoon!\n"; else if(op==3) cout<<"Good night!\n"; else cout<<"Invalid entry\n"; } int main(int lista) { menu(); welcome(); cout<<"Shuting down...\n"; return 0; }Note that it has three functions in the code:
- menu()
- welcome ()
- main ()
main() is a special function of C ++, it is automatically executed when we compile and execute our code. Inside it, we first call menu() and only when it finishes executing, it calls welcome().
Note that only after the user enters the value in welcome() and she greets you, will it end and return to main(), which displays a final closing cout.
When to use a function in C++
Ever. As much as you can.So far in our course we have done small and simple code examples.
But when you become a professional programmer, it is normal to make systems with hundreds or thousands of lines of code, and that's when you will understand how important the concept of functions is in C++.
You should get into the habit of creating small, simple, easy-to-understand and easy-to-use functions, because whenever you start a new project, you'll use your old functions and existing C++ functions by default.
This technique is called divide and conquer, and throughout our C ++ course you will see that a large and complex program is nothing more than a bunch of small, simple, correctly connected programs that work in harmony.
No comments:
Post a Comment