Exponentiation in Matemática
We call exponentiation, the mathematical operation that has two numbers: the base and the exponent.It is the famous 'x raised to y'.
For example:
3² (3 raised to the power 2, or 3 squared) - 3 is base and 2 the exponent
4³ (4 raised to the power 3, or 4 cube) - 4 is the base and 3 the exponent.
Now calculating these operations:
3² = 3 * 3 = 9
4³ = 4 * 4 * 4 = 64
5⁴ = 5 * 5 * 5 * 5 = 625
Notice how the base value repeats as often as the exponent value ... repeats ... huuuum ... repetition ... remember what? Huuum, loopings!
Exponentiation with loopings in C++
If we have a value:a ^ b (a raised to the power b), this means that the value of a will repeat b times:
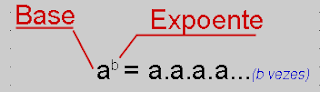
Let's ask the user for base and expo variables.
The result, let's store in res, this value is initialized to 1, because we will do a series of multiplications on this variable.
Within the FOR loop, we have an aux auxiliary variable that will count from 1 to expo, to perform expo repetitions, do you agree?
See how our code is using FOR loop:
#include <iostream> using namespace std; int main() { int base, expo, res=1, aux; cout << "Base: "; cin >> base; cout << "Exponent: "; cin >> expo; for(aux=1 ; aux<=expo ; aux++) res *= base; cout <<base<<"^"<<expo<<" = "<<res<<endl; return 0; }Now with looping WHILE:
#include <iostream> using namespace std; int main() { int base, expo, res=1, aux=1; cout << "Base: "; cin >> base; cout << "Exponent: "; cin >> expo; while(aux<=expo){ res *= base; aux++; } cout <<base<<"^"<<expo<<" = "<<res<<endl; return 0; }And finally, with the DO WHILE repeating structure (just enter 0 and / or 0 to end the calculations):
#include <iostream> using namespace std; int main() { int base, expo, res, aux; do{ cout << "Base: "; cin >> base; cout << "Expoente: "; cin >> expo; res=1; for(aux=1 ; aux<=expo ; aux++) res *= base; cout <<base<<"^"<<expo<<" = "<<res<<endl; cout<<endl; }while(base || expo); return 0; }Obviously, this algorithm works only for integer exponents, for decimals, the hole is lower.
But note that the cool, usefulness and versatility of repetition structures, our beloved loops, even serve to do mathematical operations.
No comments:
Post a Comment