WHILE Loop: Repetition Structure
As the name may suggest in the introduction, it is a structure that allows us to repeat certain pieces of code in C++.The structure of C ++ is as follows:
while(test){ // Statement in case of // test expression be true }So it starts with the while statement, then parentheses, and within these must have some boolean value, usually an expression, a conditional test that will return true or false.
While that value within parentheses is true, the code inside the while statement will run.
Each repetition is called an iteration. There can be none, one, one thousand, one million or infinite iterations in a loop (infinite loopings), it will depend on how the conditional test behaves.
See the WHILE loop flowchart:
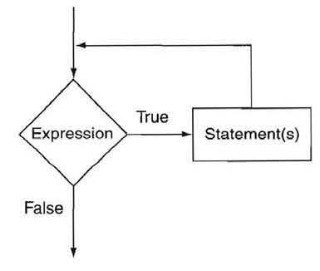
WHILE Example - Repetition Structure
Let's go to the examples, so we learn better.Let's create a program that displays numbers from 1 to 10 on the screen.
For this, let's use a control variable, let's call count, because it will be our counter.
We start with value 1.
The conditional test is: as long as count is less than or equal to 10
Inside WHILE we give cout to print the number.
Then we have to increment the counter by one, see the code:
#include <iostream> using namespace std; int main() { int count=1; while(count<=10){ cout << count << endl; count++; } return 0; }What happens is as follows. The code arrives in WHILE, and there it tests if the variable is less than or equal to 10.
As it is worth 1, the test is true and enters the code. There we print the variable, and increment the counter.
Then the test occurs again, now our variable is worth 2 and gives true to the test, running its code.
Then the test occurs with the counter worth 3, and so on, until the counter is 10.
In this case, the test is still true.
But within the code, the counter increments and turns 11.
When retesting, the result is false, so the repeat structure stops the iterations and ends the loop.
Here's what the code would look like, displaying from 10 to 1:
#include <iostream> using namespace std; int main() { int count=10; while(count>0){ cout << count << endl; count--; } return 0; }
How to use WHILE loop in C ++
The example below is infinite looping, but the test inside the WHILE loop is always true, so he would be printing the message endlessly ...#include <iostream> using namespace std; int main() { while(1) cout << "Progressive C++ course\n"; return 0; }We can also do an infinite count:
#include <iostream> using namespace std; int main() { int count=1; while(count++) cout << count << endl; return 0; }Note that we place the increment operator directly inside the while loop.
We also do not use braces, this is allowed as the while code has only one line.
WHILE in C ++: Validating Entries
One of the features of the WHILE command is to validate user input.For example, let's ask the user for a grade from 0 to 10.
If it type less than 0 or greater than 10, it will enter WHILE.
Within the repeating structure, we warn him that he entered a wrong note and we ask him to insert the note again.
The while loop is a stubborn loop ... it repeats as many times as necessary.
Want to see? Test placing notes less than 0 or greater than 10, it will only stop when the entered note is between 0 and 10:
#include <iostream> using namespace std; int main() { int grade; cout << "Type a grade: "; cin >> grade; while(grade<0 || grade>10){ cout <<"Invalid grade, try again: "; cin >> grade; } return 0; }Very simple, but powerful, this control structure.
Example of using WHILE in C ++
Create a program that prompts the user for a number and displays the multiplication table of that number.Let's store the number entered in variable num. Our control variable is aux, which will range from 1 to 10.
See how our code looks:
#include <iostream> using namespace std; int main() { int num, aux=1; cout << "Type a number: "; cin >> num; while(aux<=10){ cout <<num<<" x "<<aux<<" = "<<num*aux<<endl; aux++; } return 0; }
No comments:
Post a Comment