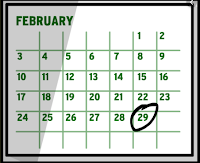
Leap years
Typically, the year has 365 days.Actually, it's a broken value, it's 365 days and 6h or 365.25 days ... but it was going to be weird a day under 24 hours, it was going to be confusing.
So to make up for those broken parts of the day, time and time we have a year with 366 days, which is February 29th.
And they occur every 4 years, minus the multiples of 100 that aren't multiples of 400.
That is, it is also leap year if it's multiple of 400.
Calm down, take a deep breath, read again ... it's a little confusing even at first.
Take a look at the history of leap years, calendars, etc., to relax a little:
https://en.wikipedia.org/wiki/Leap_year
Basically, every 4 years we have leap years:
...1996, 2000, 2004, 2008, 2012, 2016, 2020, 2024, 2028, 2032, 2036 ...
Every 100 years we don't leap year ...:
100, 200, 300 ... are not leap years
... except if they are multiples of 400:
400, 800, 1200, 1600 ... are leap years
Like this:
...
400 - is leap
500 - not leap
600 - not leap
700 - not leap
800 - is leap
...
1200 - is leap
1300 - not leap
1400 - not leap
1500 - not leap
1600 - is leap
1700 - not leap
1800 - not leap
1900 - not leap
2000 - is leap
2100 - not leap
2200 - not leap
2300 - not leap
2400 - is leap
...
Leap year code in C++
Ok, let's put this into practice by creating C ++ code.A difficult problem is nothing more than several easy problems.
So let's break this algorithm into a smaller, simpler one.
First, let's look at the multiple years of 400. If it's a multiple of 400, it's gone, it's just success, it's leap, and it's over:
And how do you verify that? Simple:
- if (year % 400 == 0)
Ready. Just say it's leap.
And if not? Well, it falls on the ELSE.
Now comes the 4 year part ... every 100 years ... that stuff weird.
Well, that's how we need to check two things:
- If is multiple of 4 (occurs every 4 years, from the year 0: 4, 8, 12, ... 1996, 2000)
- If not multiple of 100
Doing this in C ++:
- Multiple of 4: (year% 4 == 0)
- Not multiple of 100: (year% 100! = 0)
Putting the two together, we have the next IF (inside the else), using the logical AND&& operator:
- (year % 4 == 0) && (year% 100 != 0)
So our code looks like this:
#include <iostream> using namespace std; int main() { int year; cout <<"Year: "; cin >> year; if(year % 400 == 0) cout << "It's leap year" << endl; else if( (year % 4 == 0) && (year % 100 != 0) ) cout << "It's leap year" << endl; else cout << "Not a leap year" << endl; return 0; }
Better Leap Year Algorithm in C++
Programmer likes to write little.
The simpler and more direct the code, the better.
Note in the previous code that we have two conditions for the year to be leap:
- (year % 400 == 0)
- ((year % 4 == 0) && (year % 100 != 0))
If one thing or another happens, it is leap.
Or ... huumm ... or remember what? Logical Operator ||
That is, we can join the two conditions and have only one IF conditional test:
- (year% 400 == 0) || ( (year% 4 == 0) && (year% 100! = 0) )
Our code looks like this:
#include <iostream> using namespace std; int main() { int year; cout <<"Year: "; cin >> year; if( (year % 400 == 0) || ( (year % 4 == 0) && (year % 100 != 0) ) ) cout << "It's leap year" << endl; else cout << "Not a leap year" << endl; return 0; }
Beautiful, huh?
No comments:
Post a Comment