Comments: What are they? What are used for? Why use?
One of the most important parts of programming code, oddly enough, is something that the compiler ignores: comments.We use comments for human communication, so you programmer can say something to another programmer who will read your code (may even yourself, in the future).
They are often used to describe in our human language what the following lines of code will do, how they work, what they are for, and give as many details as possible.
It may seem silly at first, and indeed it is, because at first our codes are very small.
But as they get bigger and more complex, it becomes hard try to understand what a code just at looking it, it would be much easier if it came with explanations, and that's where comments come in.
Imagine you programmed a C++ game, tens of thousands of lines, launched, got rich and everything. However, 5 months later, people discovered a bug.
Bro, you can be sure: when reviewing the code, you will have extreme difficulty knowing what each excerpt does and what its function, we really forget and it takes an absurd job trying to understand a code just seeing that alphabet soup.
Comment on your codes, explain what they are, what they are for, what a particular logic or algorithm is doing, and give as much information as possible. This is an excellent programming habit: commenting on codes.
How to comment a line in C++: //
To comment a line (that is, make a line of code a comment), simply start it with two bars:#include <iostream> using namespace std; int main() { // Rush is the best band ever cout << "Welcome to Progressive C++ website"; return 0; }
Note that the text "Rush is the best band in the world" does not appear on the user's screen:
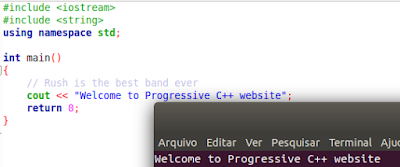
Let's take a really useful example of code. The program below calculates the area of a circle:
#include <iostream> using namespace std; int main() { float rad, area; // Ask for radius cout << "Radius value: "; cin >> rad; // Calculating the area area = 3.14 * rad * rad; cout << "Area: " << area; return 0; }
Remember, it may sound silly now, but it's because they are simple examples.
As our algorithms get bigger and more complex, you'll notice the importance of commenting on your code.
It is a clear feature present in the best programmers, and as you have studied by Progressive C ++, it is certainly part of this select group. So, COMMENT YOUR CODES.
How to comment multiple lines: /* */
It's often interesting that we use multiple lines to correctly comment a code.This is quite common at the beginning of files, giving a brief description of the program, its functions, speaking of the author, etc.
That would be a lot of work using the // bars in every line.
To comment multiple lines at once, start with: / *
Write what you want.
End with: * /
Anything between these two symbols is considered a comment.
Let's make a C ++ program that asks for the radius, and shows the value of the perimeter and area of a circle.
/* Program: circle.cpp Author: Progressive C++ Objective: this program receives the radius value, returns the perimeter value and the area of the circle */ #include <iostream> using namespace std; int main() { float rad, area, perim; cout << "Radius: "; cin >> rad; area = 3.14 * rad * rad; perim = 2 * 3.14 * rad; cout << "Perimeter: " << perim << endl; cout << "Area: " << area; return 0; }
No comments:
Post a Comment